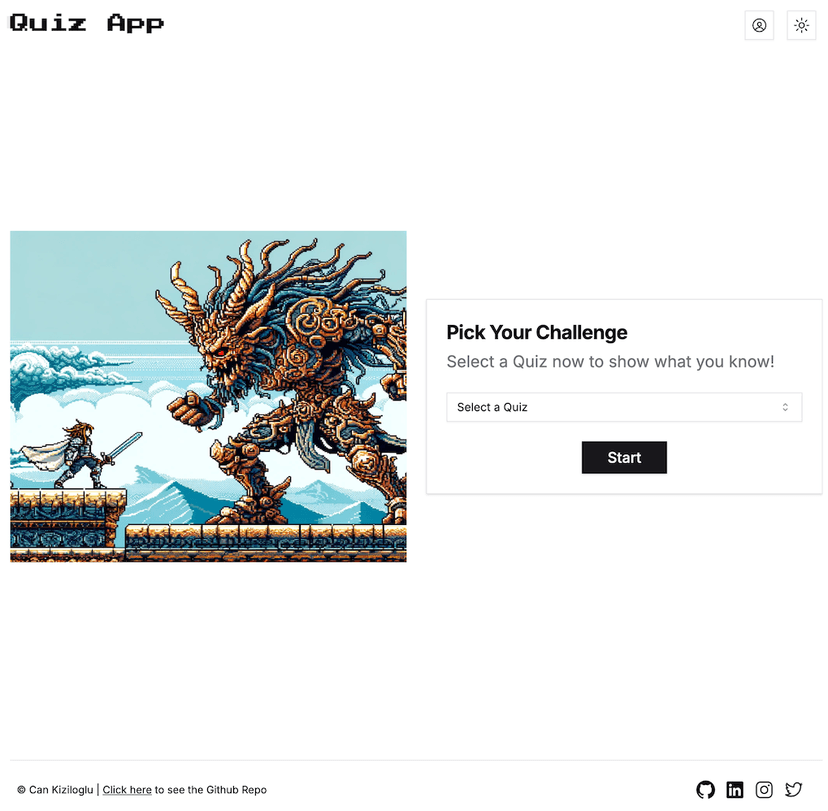
Quiz App
This is a full-stack Quiz Application built with Node.js, Express, React, and Tailwind CSS. The app allows users to take quizzes, view their attempts, and manage quizzes and questions.
Tech Stack
Backend
- Node.js: JavaScript runtime for building server-side applications.
- Express: Web framework for Node.js.
- Prisma:
- Joi: Data validation library.
- Joi-password-complexity: Password complexity validation for Joi.
- dotenv: Loads environment variables from a
.env
file. - helmet: Security middleware for Express.
- morgan: HTTP request logger middleware for Node.js.
- cors: Middleware for enabling Cross-Origin Resource Sharing.
- cookie-parser: Middleware for parsing cookies.
Frontend
- React: JavaScript library for building user interfaces.
- React Router: Declarative routing for React.
- Tailwind CSS: Utility-first CSS framework.
- React Query: Data-fetching library for React.
- TypeScript: Typed superset of JavaScript.
- Vite: Next-generation frontend tooling.
Features
Backend
- User authentication and authorization.
- Quiz management (create, edit, delete quizzes).
- Question management (create, edit, delete questions).
- Attempt management (start and submit, view and delete attempts).
- Data validation using Joi.
- Database queries and transactions using Prisma ORM.
Frontend
- User login, logout and signup.
- Quiz taking interface, one question at a time.
- View & edit account info & password.
- View past quiz attempts and scores.
- Responsive design with Tailwind CSS.
- State management using React Context API.
- Admin dashboard to manage quizzes, questions, answers, attempts and users.
Deployment
I deployed this app using an AWS EC2 instance running Ubuntu. I didn't containerize the app, instead pulled the repository from github and built it on the server and served it using pm2 on the backend and nginx on the frontend.
How the App Works
Backend
The backend is built with Node.js and Express. It provides RESTful APIs for managing users, quizzes, questions, and attempts. Data validation is handled using Joi. Database queries and transactions are handled with Prisma Orm using a Postgres database. The backend includes middleware for authentication, authorization, security, logging, and error handling. User passwords are hashed using bcrypt and session tokens are created using jwt and passed as cookies to the frontend.
When a user requests to take a quiz, a new attempt is created in the database. Questions are fetched from the db and shuffled and sliced according to the quiz length for the selected quiz and attached to the attempt sent to the frontend. When the user completes the quiz the attempt is updated with user answers and the score is calculated and sent back to the frontend.
Frontend
The frontend is built with React and TypeScript. It uses React Router for navigation and React Query for data fetching. The UI is styled using Tailwind CSS. The frontend communicates with the backend APIs to perform CRUD operations and manage user sessions. When a user starts an attempt to a quiz, the questions are stored in local storage, so if the user terminates that session and comes back later to solve the same quiz, questions are served from local storage. Authentication, quiz and attempt data are managed using state (context API).
When the user finishes and submits a quiz the result is fetched from the backend and shown to the user. User can also view past attempts and their scores and details in their account page.
Admins can manage (view, create, edit, delete) users, quizzes, questions and attempts on their dashboard.
Details
Why build this app?
This app has an intensive use of database tables and relations. There are lots of api endpoints to create / read / update / delete quizzes, questions with their answers, users and quiz attempts. This app also utilizes authentication and authorization built from scratch using cookies, json web tokens and password hashing.
Also, quizzes are fun!
What were the challenges and how did I overcome them?
Database design was the first challenge, including creating a correct entity relationship diagram and turning it into a prisma schema. I overcame this challenge by carefully planning the features of the app from the start without any coding. This enabled me to design the database and endpoints before starting to code.
Creating all the necessary api endpoints was another challenge. But using express and prisma it was rather easy.
Building the frontend was more challenging than the backend. After deciding on how to handle state, implementing Context API together with local storage was rather easy.
Admin dashboards required lots of tables and database calls. I managed to create a single data table component and use it for all tables.
Links
Note: In order to take quizzes you have to login. You can sign up with some made up email and password, there is no email confirmation scenario. If you want to access admin dashboard and see its functionality however, you have to be assigned an admin role by an admin. Just email me and I can help you with that.